Building a MLM for clothing using Tensorflow and Keras (NOT FINISHED)
This project was inspired by my interest in Japanese/Korean fashion brands online, but never being able to know the brand/design.
This application was build entirely in Python using opencv, tensorflow/keras, and customtkinter. Using CNNs (convolutional neural networks), I created a pre-trained ResNet50 model using a dataset I created (via pinterest).
Logic/Steps
1. Data collection and organization
As an initial test, I added two brands: Stussy and Chrome Hearts. I collected the images off of pinterest and added them to their respective folders within a raw dataset.
Since all the images came in different sizes/formats, I created a super short script that automates processing to save me time:
import os from PIL import Image # Constants IMG_SIZE = (224, 224) IMAGE_FORMAT = 'JPEG' def preprocess_image(image_path, save_path): with Image.open(image_path) as img: # Resize the image to (224, 224) img = img.resize(IMG_SIZE) # Convert the image to RGB format img = img.convert('RGB') # Save the processed image img.save(save_path, format=IMAGE_FORMAT) print(f'Processed image saved to {save_path}') # For debugging def preprocess_directory(input_dir, output_dir): # Ensure output directory exists os.makedirs(output_dir, exist_ok=True) for root, _, files in os.walk(input_dir): for filename in files: if filename.lower().endswith(('.jpg', '.jpeg', '.png')): # Check if the file is an image brand = os.path.basename(root) brand_output_dir = os.path.join(output_dir, brand) os.makedirs(brand_output_dir, exist_ok=True) input_path = os.path.join(root, filename) output_path = os.path.join(brand_output_dir, filename) preprocess_image(input_path, output_path)
2. Model Training
Using Tensorflow/Keras, I pre-trained a ResNet50 model, and fine-tuned it with custom layers to adapt to the specific task of ID recognition.
I implemented a script as well which essentially just evaluated test loss and accuracy
First eval:

So... not very good
At it's first run, the model achieved a 50% test accuracy, which is paramount to just random guessing, but this is mainly due to the small sample size of images I started off with. Ideally, having 500-600 images per brand/ID is ideal, and I started off with around 15 each to start. My main struggle here is finding a way to automate gathering the hundreds of images off of pinterest/grailed.
3. Creating the GUI
Next, I used customtkinter to create a GUI where the user can select an image off of their computer to evaluate, which worked perfectly.
Here's a screenshot of the GUI:
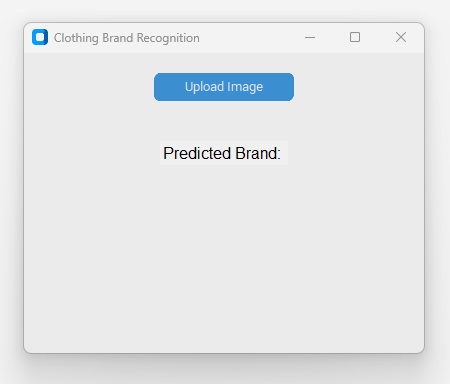
The user selects an image, and the model is evaluated, and the result is displayed.
4. Future Work
There is still a lot more work to be done, and I'll gradually update this as I work more on it, but these are the key aspects I plan on implementing:
Technologies Used
- Python
- Tensorflow
- Keras
- Customtkinter
- PIL
Sources
N/A